Command Line Parameter Regex July, 2009
Recently I needed a regex to parse the command line. Something like this:
/param1="sfsdf sdf sdfsdf sdfsdf" /param2 =rstwertw /param3 = "rtwqert sdfas f"
I found a great regex on RegExLib by Richard Hauer for doing this. I made one minor tweak to allow whitespace between the name/value delimiter:
(?:\s*)(?<=[-|/])(?
Here it is in action:
string commandLineRegEx = @"(?:\s*)(?<=[-|/])(?\w*)\s*[:|=]\s*(""((? ; Regex regex = new Regex(commandLineRegEx); MatchCollection matches = regex.Matches( "/param1=\"sfsdf sdf sdfsdf sdfsdf\" /param2 =rstwertw /param3 = \"rtwqert sdfas f\""); foreach (Match match in matches) Console.WriteLine("{0}={1}", match.Groups["name"].Value, match.Groups["value"].Value); Console.ReadKey();.*?)(?[\w]*))"
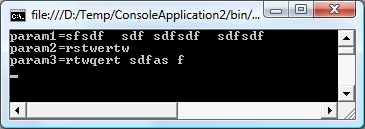